How to create an ERC-4337 Smart Wallet and send a Sponsored Transaction
Smart Wallets powered by the ERC-4337 standard are a new way of representing wallets on EVM-compatible blockchain networks.
In contrast to traditional wallets based on a private key (aka EOAs), smart wallets provide new exciting features, like the ability to sign/authenticate transactions with a different mechanism than just a private key, the ability to sponsor the gas required to run a transaction, the ability to have social login, recovery, plugins, basically anything that you can program with Solidity.
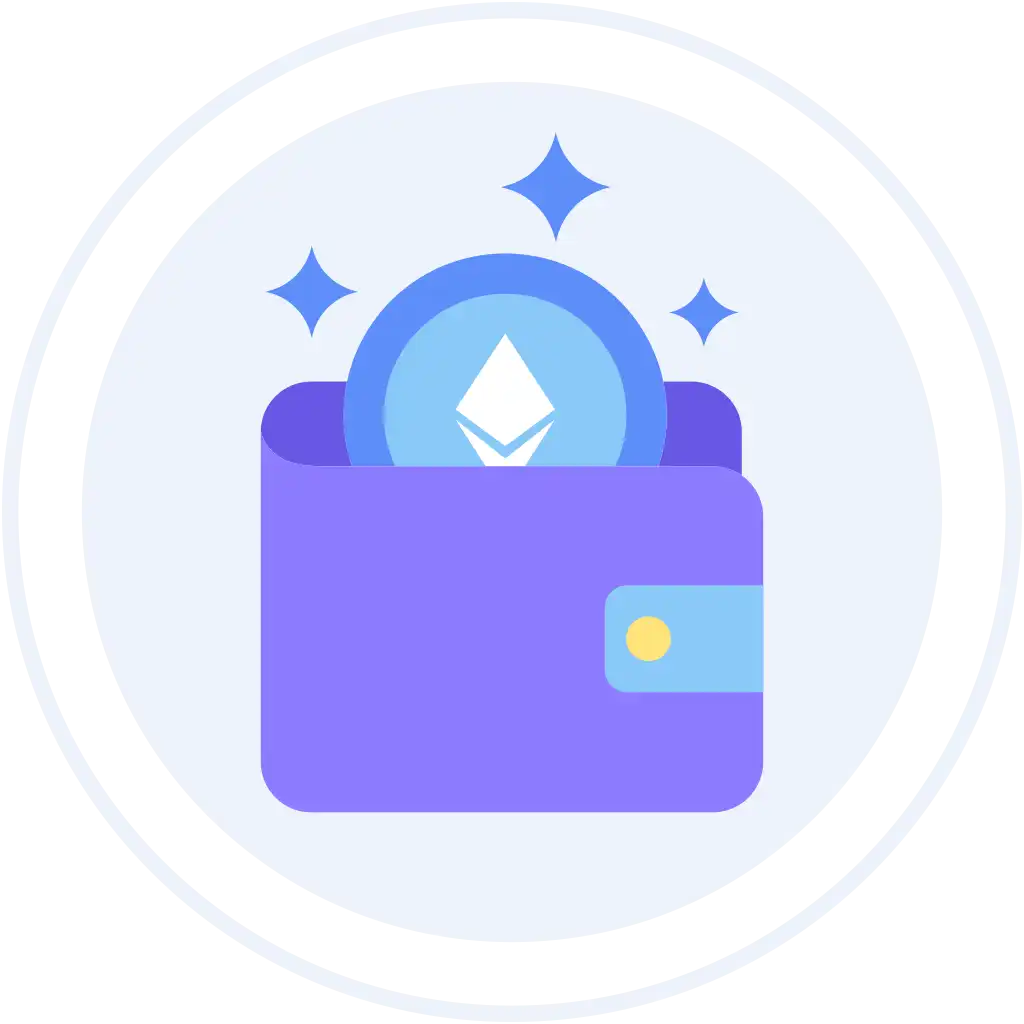
How to create an ERC-4337 Smart Wallet?
The first step in creating a smart wallet is deciding which smart wallet implementation you want to use. There are a few popular choices like:
In this article, we will be using the ZeroDev Kernel.
Apart from the smart wallet implementation, you will need a few more things:
- An SDK to create and interact with the smart wallet
- An RPC/Bundler endpoint
- A Paymaster
As an SDK, we are going to use the Kriptonio SDK which also automatically creates the rpc, bundler, and paymaster endpoints for us.
Coding time
The first step is to install Kriptonio SDK:
yarn add @kriptonio/sdk
If you are a Typescript user, we suggest that you check the additional setup hints here.
Next, you will need to acquire an access token for the Kriptonio SDK. Here is how:
- Go to Kriptonio Web App
- Create an account
- Go to the settings page (top right corner, cog icon)
- Select the
Access Tokens
tab and copy your access token
Now we are ready to initialize the Kriptonio SDK:
import { ChainId, KriptonioSdk } from '@kriptonio/sdk';
const sdk = new KriptonioSdk({ accessToken: 'paste-access-token-here' });
With the Kriptonio SDK, you can manage both smart and classic wallets. Smart wallets are the default, so to create one,
we will use the wallet generate
function. The only thing you need to provide is the wallet type and chain id of the
chain with which you want to interact.
const wallet = await sdk.wallet.generate({
chainId: ChainId.BaseSepolia,
type: 'kernel',
});
Congrats, you have created an ERC-4337 smart wallet! 🎉.
Under the hood, the Kriptonio SDK creates a smart wallet powered by the ZeroDev Kernel. Now you can use the wallet
object to check the wallet address.
console.log(wallet.address);
It's now time to send a transaction using your smart wallet.
const hash = await wallet.sendTransaction({
to: wallet.address,
value: 0n,
});
That's it! You have created a smart wallet and sent a transaction using it.
But what happened under the hood?
Under the hood, the Kriptonio SDK created the RPC, Bundler, and Paymaster endpoints. It also sponsored the transaction gas fee for you, so you didn't have to worry about it.
Another thing that happened is that since this was the first transaction from your smart wallet, it has been automatically deployed to the selected blockchain network, in this case, the Base Sepolia network.
You did great! To learn more about smart wallets and how to utilize the Kriptonio SDK more, check out the official docs.
If you need help, feel free to reach us via our Discord server https://discord.gg/7QreJNYuaz.
Source Code
The complete source code for this article can be found here.
Bonus Tip
You can use the Kriptonio iOS/Android mobile apps to import smart wallets that you created with the Kriptonio SDK.
Links to the mobile apps are here: https://docs.kriptonio.com/mobile